Bayesian Parametric Survival Analysis with PyMC3
Survival analysis studies the distribution of the time between when a subject comes under observation and when that subject experiences an event of interest. One of the fundamental challenges of survival analysis (which also makes it mathematically interesting) is that, in general, not every subject will experience the event of interest before we conduct our analysis. In more concrete terms, if we are studying the time between cancer treatment and death (as we will in this post), we will often want to analyze our data before every subject has died. This phenomenon is called censoring and is fundamental to survival analysis.
I have previously written about Bayesian survival analysis using the semiparametric Cox proportional hazards model. Implementing that semiparametric model in PyMC3 involved some fairly complex numpy
code and nonobvious probability theory equivalences. This post illustrates a parametric approach to Bayesian survival analysis in PyMC3. Parametric models of survival are simpler to both implement and understand than semiparametric models; statistically, they are also more powerful than non- or semiparametric methods when they are correctly specified. This post will not further cover the differences between parametric and nonparametric models or the various methods for chosing between them.
As in the previous post, we will analyze mastectomy data from R
’s HSAUR
package. First, we load the data.
%matplotlib inline
from matplotlib import pyplot as plt
from matplotlib.ticker import StrMethodFormatter
import numpy as np
import pymc3 as pm
import scipy as sp
import seaborn as sns
from statsmodels import datasets
from theano import shared, tensor as tt
set()
sns.= sns.color_palette()
blue, green, red, purple, gold, teal
= StrMethodFormatter('{x:.1%}') pct_formatter
= (datasets.get_rdataset('mastectomy', 'HSAUR', cache=True)
df
.data=lambda df: 1. * (df.metastized == "yes"),
.assign(metastized=lambda df: 1. * df.event)) event
df.head()
time | event | metastized | |
---|---|---|---|
0 | 23 | 1.0 | 0.0 |
1 | 47 | 1.0 | 0.0 |
2 | 69 | 1.0 | 0.0 |
3 | 70 | 0.0 | 0.0 |
4 | 100 | 0.0 | 0.0 |
The column time
represents the survival time for a breast cancer patient after a mastectomy, measured in months. The column event
indicates whether or not the observation is censored. If event
is one, the patient’s death was observed during the study; if event
is zero, the patient lived past the end of the study and their survival time is censored. The column metastized
indicates whether the cancer had metastized prior to the mastectomy. In this post, we will use Bayesian parametric survival regression to quantify the difference in survival times for patients whose cancer had and had not metastized.
Accelerated failure time models
Accelerated failure time models are the most common type of parametric survival regression models. The fundamental quantity of survival analysis is the survival function; if \(T\) is the random variable representing the time to the event in question, the survival function is \(S(t) = P(T > t)\). Accelerated failure time models incorporate covariates \(\mathbf{x}\) into the survival function as
\[S(t\ |\ \beta, \mathbf{x}) = S_0\left(\exp\left(\beta^{\top} \mathbf{x}\right) \cdot t\right),\]
where \(S_0(t)\) is a fixed baseline survival function. These models are called “accelerated failure time” because, when \(\beta^{\top} \mathbf{x} > 0\), \(\exp\left(\beta^{\top} \mathbf{x}\right) \cdot t > t\), so the effect of the covariates is to accelerate the effective passage of time for the individual in question. The following plot illustrates this phenomenon using an exponential survival function.
= sp.stats.expon.sf S0
= plt.subplots(figsize=(8, 6))
fig, ax
= np.linspace(0, 10, 100)
t
5 * t),
ax.plot(t, S0(=r"$\beta^{\top} \mathbf{x} = \log\ 5$");
label2 * t),
ax.plot(t, S0(=r"$\beta^{\top} \mathbf{x} = \log\ 2$");
label
ax.plot(t, S0(t),=r"$\beta^{\top} \mathbf{x} = 0$ ($S_0$)");
label0.5 * t),
ax.plot(t, S0(=r"$\beta^{\top} \mathbf{x} = -\log\ 2$");
label0.2 * t),
ax.plot(t, S0(=r"$\beta^{\top} \mathbf{x} = -\log\ 5$");
label
0, 10);
ax.set_xlim(r"$t$");
ax.set_xlabel(
;
ax.yaxis.set_major_formatter(pct_formatter)-0.025, 1);
ax.set_ylim(r"Survival probability, $S(t\ |\ \beta, \mathbf{x})$");
ax.set_ylabel(
=1);
ax.legend(loc"Accelerated failure times"); ax.set_title(
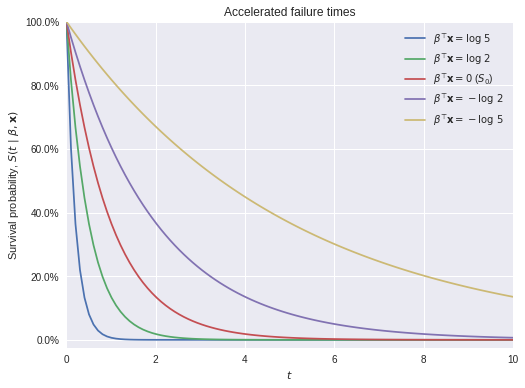
Accelerated failure time models are equivalent to log-linear models for \(T\),
\[Y = \log T = \beta^{\top} \mathbf{x} + \varepsilon.\]
A choice of distribution for the error term \(\varepsilon\) determines baseline survival function, \(S_0\), of the accelerated failure time model. The following table shows the correspondence between the distribution of \(\varepsilon\) and \(S_0\) for several common accelerated failure time models.
Log-linear error distribution (\(\varepsilon\)) | Baseline survival function (\(S_0\)) |
---|---|
Normal | Log-normal |
Extreme value (Gumbel) | Weibull |
Logistic | Log-logistic |
Accelerated failure time models are conventionally named after their baseline survival function, \(S_0\). The rest of this post will show how to implement Weibull and log-logistic survival regression models in PyMC3 using the mastectomy data.
Weibull survival regression
In this example, the covariates are \(\mathbf{x}_i = \left(1\ x^{\textrm{met}}_i\right)^{\top}\), where
\[ \begin{align*} x^{\textrm{met}}_i & = \begin{cases} 0 & \textrm{if the } i\textrm{-th patient's cancer had not metastized} \\ 1 & \textrm{if the } i\textrm{-th patient's cancer had metastized} \end{cases}. \end{align*} \]
We construct the matrix of covariates \(\mathbf{X}\).
= df.shape
n_patient, _
= np.empty((n_patient, 2))
X 0] = 1.
X[:, 1] = df.metastized X[:,
We place independent, vague normal prior distributions on the regression coefficients,
\[\beta \sim N(0, 5^2 I_2).\]
= 5. VAGUE_PRIOR_SD
with pm.Model() as weibull_model:
= pm.Normal('β', 0., VAGUE_PRIOR_SD, shape=2) β
The covariates, \(\mathbf{x}\), affect value of \(Y = \log T\) through \(\eta = \beta^{\top} \mathbf{x}\).
= shared(X)
X_
with weibull_model:
= β.dot(X_.T) η
For Weibull regression, we use
\[ \begin{align*} \varepsilon & \sim \textrm{Gumbel}(0, s) \\ s & \sim \textrm{HalfNormal(5)}. \end{align*} \]
with weibull_model:
= pm.HalfNormal('s', 5.) s
We are nearly ready to specify the likelihood of the observations given these priors. Before doing so, we transform the observed times to the log scale and standardize them.
= np.log(df.time.values)
y = (y - y.mean()) / y.std() y_std
The likelihood of the data is specified in two parts, one for uncensored samples, and one for censored samples. Since \(Y = \eta + \varepsilon\), and \(\varepsilon \sim \textrm{Gumbel}(0, s)\), \(Y \sim \textrm{Gumbel}(\eta, s)\). For the uncensored survival times, the likelihood is implemented as
= df.event.values == 0. cens
= shared(cens)
cens_
with weibull_model:
= pm.Gumbel(
y_obs 'y_obs', η[~cens_], s,
=y_std[~cens]
observed )
For censored observations, we only know that their true survival time exceeded the total time that they were under observation. This probability is given by the survival function of the Gumbel distribution,
\[P(Y \geq y) = 1 - \exp\left(-\exp\left(-\frac{y - \mu}{s}\right)\right).\]
This survival function is implemented below.
def gumbel_sf(y, μ, σ):
return 1. - tt.exp(-tt.exp(-(y - μ) / σ))
We now specify the likelihood for the censored observations.
with weibull_model:
= pm.Bernoulli(
y_cens 'y_cens', gumbel_sf(y_std[cens], η[cens_], s),
=np.ones(cens.sum())
observed )
We now sample from the model.
= 845199 # from random.org, for reproducibility
SEED
= {
SAMPLE_KWARGS 'njobs': 3,
'tune': 1000,
'random_seed': [
SEED,+ 1,
SEED + 2
SEED
] }
with weibull_model:
= pm.sample(**SAMPLE_KWARGS) weibull_trace
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
100%|██████████| 1500/1500 [00:04<00:00, 322.90it/s]
The energy plot and Bayesian fraction of missing information give no cause for concern about poor mixing in NUTS.
; pm.energyplot(weibull_trace)
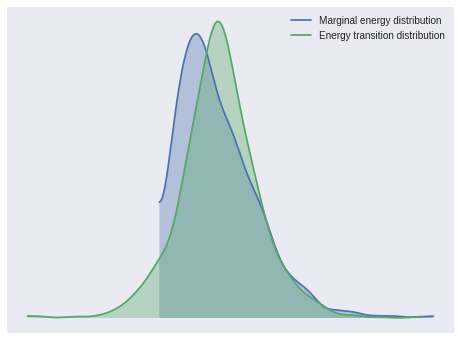
pm.bfmi(weibull_trace)
1.0189285246960067
The Gelman-Rubin statistics also indicate convergence.
max(np.max(gr_stats) for gr_stats in pm.gelman_rubin(weibull_trace).values())
1.0077500079163573
Below we plot posterior distributions of the parameters.
=0, alpha=0.5); pm.plot_posterior(weibull_trace, lw
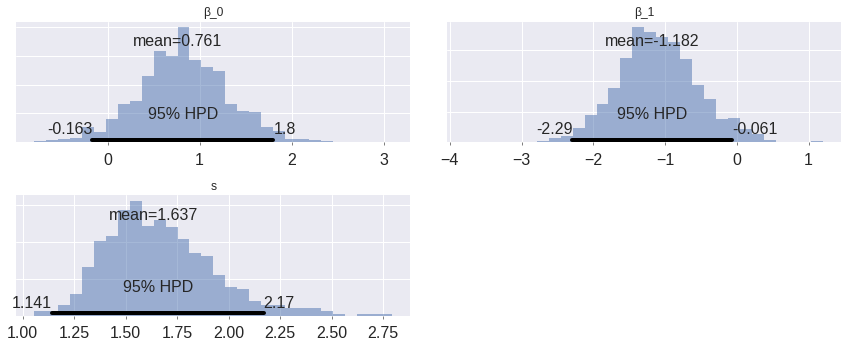
These are somewhat interesting (espescially the fact that the posterior of \(\beta_1\) is fairly well-separated from zero), but the posterior predictive survival curves will be much more interpretable.
The advantage of using theano.shared
variables is that we can now change their values to perform posterior predictive sampling. For posterior prediction, we set \(X\) to have two rows, one for a subject whose cancer had not metastized and one for a subject whose cancer had metastized. Since we want to predict actual survival times, none of the posterior predictive rows are censored.
= np.empty((2, 2))
X_pp 0] = 1.
X_pp[:, 1] = [0, 1]
X_pp[:,
X_.set_value(X_pp)
= np.repeat(False, 2)
cens_pp cens_.set_value(cens_pp)
with weibull_model:
= pm.sample_ppc(
pp_weibull_trace =1500, vars=[y_obs]
weibull_trace, samples )
100%|██████████| 1500/1500 [00:00<00:00, 2789.50it/s]
The posterior predictive survival times show that, on average, patients whose cancer had not metastized survived longer than those whose cancer had metastized.
= np.linspace(0, 230, 100)
t_plot
= (np.greater_equal
weibull_pp_surv + y.std() * pp_weibull_trace['y_obs']),
.outer(np.exp(y.mean()
t_plot))= weibull_pp_surv.mean(axis=0) weibull_pp_surv_mean
= plt.subplots(figsize=(8, 6))
fig, ax
0],
ax.plot(t_plot, weibull_pp_surv_mean[=blue, label="Not metastized");
c1],
ax.plot(t_plot, weibull_pp_surv_mean[=red, label="Metastized");
c
0, 230);
ax.set_xlim("Weeks since mastectomy");
ax.set_xlabel(
=1);
ax.set_ylim(top;
ax.yaxis.set_major_formatter(pct_formatter)"Survival probability");
ax.set_ylabel(
=1);
ax.legend(loc"Weibull survival regression model"); ax.set_title(
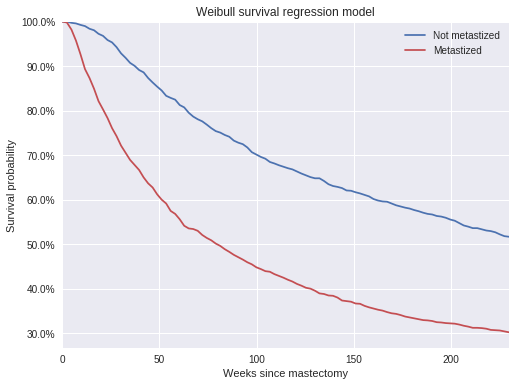
Log-logistic survival regression
Other accelerated failure time models can be specificed in a modular way by changing the prior distribution on \(\varepsilon\). A log-logistic model corresponds to a logistic prior on \(\varepsilon\). Most of the model specification is the same as for the Weibull model above.
X_.set_value(X)
cens_.set_value(cens)
with pm.Model() as log_logistic_model:
= pm.Normal('β', 0., VAGUE_PRIOR_SD, shape=2)
β = β.dot(X_.T)
η
= pm.HalfNormal('s', 5.) s
We use the prior \(\varepsilon \sim \textrm{Logistic}(0, s)\). The survival function of the logistic distribution is
\[P(Y \geq y) = 1 - \frac{1}{1 + \exp\left(-\left(\frac{y - \mu}{s}\right)\right)},\]
so we get the likelihood
def logistic_sf(y, μ, s):
return 1. - pm.math.sigmoid((y - μ) / s)
with log_logistic_model:
= pm.Logistic(
y_obs 'y_obs', η[~cens_], s,
=y_std[~cens]
observed
)= pm.Bernoulli(
y_cens 'y_cens', logistic_sf(y_std[cens], η[cens_], s),
=np.ones(cens.sum())
observed )
We now sample from the log-logistic model.
with log_logistic_model:
= pm.sample(**SAMPLE_KWARGS) log_logistic_trace
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
100%|██████████| 1500/1500 [00:05<00:00, 291.48it/s]
All of the sampling diagnostics look good for this model.
; pm.energyplot(log_logistic_trace)
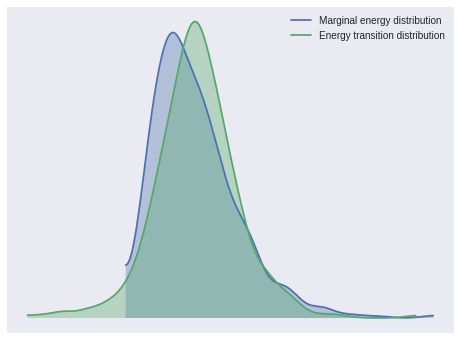
pm.bfmi(log_logistic_trace)
0.98805328946082049
max(np.max(gr_stats) for gr_stats in pm.gelman_rubin(log_logistic_trace).values())
1.0018938145216476
Again, we calculate the posterior expected survival functions for this model.
X_.set_value(X_pp)
cens_.set_value(cens_pp)
with log_logistic_model:
= pm.sample_ppc(
pp_log_logistic_trace =1500, vars=[y_obs]
log_logistic_trace, samples )
100%|██████████| 1500/1500 [00:00<00:00, 2526.82it/s]
= (np.greater_equal
log_logistic_pp_surv + y.std() * pp_log_logistic_trace['y_obs']),
.outer(np.exp(y.mean()
t_plot))= log_logistic_pp_surv.mean(axis=0) log_logistic_pp_surv_mean
= plt.subplots(figsize=(8, 6))
fig, ax
0],
ax.plot(t_plot, weibull_pp_surv_mean[=blue, label="Weibull, not metastized");
c1],
ax.plot(t_plot, weibull_pp_surv_mean[=red, label="Weibull, metastized");
c
0],
ax.plot(t_plot, log_logistic_pp_surv_mean['--', c=blue,
="Log-logistic, not metastized");
label1],
ax.plot(t_plot, log_logistic_pp_surv_mean['--', c=red,
="Log-logistic, metastized");
label
0, 230);
ax.set_xlim("Weeks since mastectomy");
ax.set_xlabel(
=1);
ax.set_ylim(top;
ax.yaxis.set_major_formatter(pct_formatter)"Survival probability");
ax.set_ylabel(
=1);
ax.legend(loc"Weibull and log-logistic\nsurvival regression models"); ax.set_title(
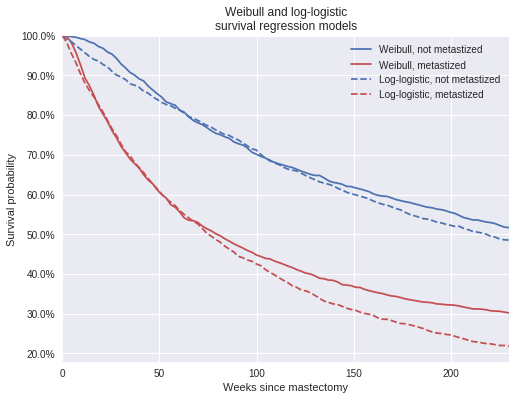
This post has been a short introduction to implementing parametric survival regression models in PyMC3 with a fairly simple data set. The modular nature of probabilistic programming with PyMC3 should make it straightforward to generalize these techniques to more complex and interesting data sets.
This post is available as a Jupyter notebook here.